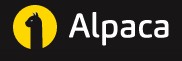
Alpaca is a US stock brokerage that allows subscribers to buy and sell stocks by calling their Application Programming Interfaces, or APIs for short. This is suitable for algorithmic traders and developers who want to automate their trading.
In the world of programming, APIs enable companies to open up their applications’ functionalities and data to external developers. In this case, Alpaca is allowing developers to use their functionalities (e.g. placing orders, viewing an account’s positions or querying the current stock price, etc.) by writing program(s) that will call to Alpaca APIs.
Before jumping in on how users can programmatically buy and sell stocks, it is equally important to learn how to get the current price of a stock or how the stock has been trading. This post focuses on a particular set of APIs that Alpaca offers — Market Data API and how can we stream the following:
Real-time Trade data of one or more stock ticker symbols
Real-time Quote data of one or more stock ticker symbols
Real-time 1-Minute bar data of one or more stock ticker symbols
The latest version of Alpaca Market Data is Market Data API v2. The API v2 provides market data in two plans: Free and Unlimited. The Free plan is included in both paper-trading and live trading accounts as the default plan. Some info from How to Stream Real-Time Data in Alpaca Market Data API v2:
* Users with Free Plan can only connect to the IEX data source.
* Users with the Unlimited Plan have direct feeds of trades, quotes, and minute bars from the CTA (administered by NYSE) and UTP (administered by Nasdaq) SIPs.
* Users with Free Plan are allowed one concurrent connection and the subscription is limited to 30 channels at a time for trades and quotes. It is worth noting that there is no limit to the number of channels with minute bars.
* Users with Unlimited Plan have no limit for the number of channels at a time for trades, quotes, and minute bars.
For this demo, we will show how to use Alpaca’s Stock Market Data API with an Alpaca Free Plan account.
Prerequisites:
The following are required before we can stream any Alpaca data:
Prerequisite #1. Install NodeJS from https://nodejs.org/en/download/
Prerequisite #2. After installing NodeJs, install wscat on the Command Prompt by executing:
C:\npm install -g wscat
Prerequisite #3. Sign up for an Alpaca account. Choose between Free and Unlimited subscription plan . I am using Free Subscription Plan.
Prerequisite #4. From Paper Trading Dashboard view, create an API. Save both API ID and Secret Key. These will be used later to authenticate your connection to Alpaca server. Note that paper trading is a real-time simulation environment but with fake money.
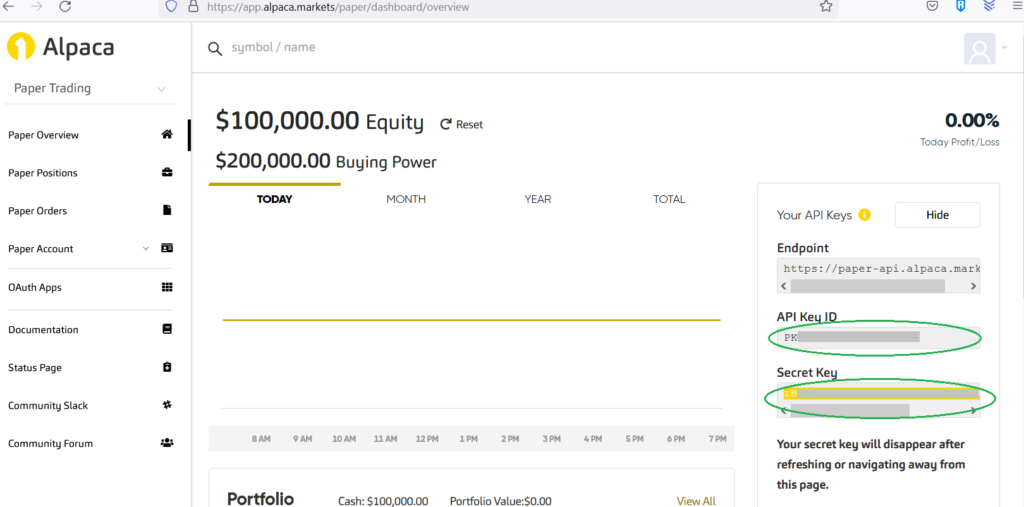
Connect to WebSocket API Stream using wscat
From the Command Prompt, enter wscat with the below format:
wscat -c wss://stream.data.alpaca.markets/v2/<SOURCE>
Where <SOURCE> depends on your Alpaca subscription. For Free plans, it will be iex. For Unlimited plans, you can use iex or sip.
Example:
wscat -c wss://stream.data.alpaca.markets/v2/iex
Success response:
< [{"T":"success","msg":"connected"}]
Authenticate using the API key and secret
After establishing a successful connection, you have to be authenticated. Enter the below in the wscat command line:
{"action": "auth", "key": "<ALPACA-API-KEY-ID>", "secret": "<ALPACA-API-SECRET-KEY>"}
Where <ALPACA-API-KEY-ID> is the API Key and <ALPACA-API-SECRET-KEY> is the Secret Key created earlier from Prerequisite #4.
Example:
{"action": "auth", "key": "PKAAAAAAAAAAAAAAAAAA", "secret": "rBaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa"}
Success response:
< [{"T":"success","msg":"authenticated"}]
Streaming Real-time Trades
If you want to stream trade data for a particular stock or stocks, enter below command from wscat command line:
{"action":"subscribe","trades":[<STOCK_SYMBOL_LIST>]}
Where <STOCK_SYMBOL_LIST> is a list of one or more stock ticker symbols.
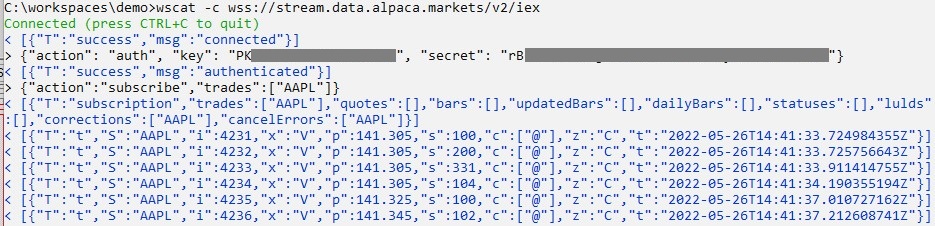
Example:
{"action":"subscribe","trades":["AAPL"]}
{"action":"subscribe","trades":["AAPL","MSFT"]}
Success response:
< [{"T":"subscription","trades":["AAPL"],"quotes":[],"bars":[],"updatedBars":[],"dailyBars":[],"statuses":[],"lulds":[],"corrections":["AAPL"],"cancelErrors":["AAPL"]}]
< [{"T":"t","S":"AAPL","i":8308,"x":"V","p":158.97,"s":100,"c":["@"],"z":"C","t":"2022-05-05T15:26:13.907398518Z"}]
Beautified version of the response:
After subscribing, you will receive a message that describes your current list of subscriptions.
{
"T": "subscription",
"trades": [
"AAPL"
],
"quotes": [],
"bars": [],
"updatedBars": [],
"dailyBars": [],
"statuses": [],
"lulds": [],
"corrections": [
"AAPL"
],
"cancelErrors": [
"AAPL"
]
}
Refer to Trade Schema
{
"T": "t",
"S": "AAPL",
"i": 8308,
"x": "V",
"p": 158.97,
"s": 100,
"c": [
"@"
],
"z": "C",
"t": "2022-05-05T15:26:13.907398518Z"
}
Trade Schema
Attribute | Type | Notes |
---|---|---|
T | string | message type, always “t” |
S | string | symbol |
i | int | trade ID |
x | string | exchange code where the trade occurred (Refer to Exchange Codes section) |
p | number | trade price |
s | int | trade size |
t | string | RFC-3339 formatted timestamp with nanosecond precision (Displayed in UTC timezone) |
c | array | trade condition |
z | string | tape (Refer to Exchange Codes section) |
Streaming Real-Time Quotes
If you want to stream quote data (i.e. the last price at which a particular stock or stocks traded), enter below command from wscat command line:
{"action":"subscribe","quotes":[<STOCK_SYMBOL_LIST>]}
Where <STOCK_SYMBOL_LIST> is a list of one or more stock ticker symbols.

Example:
{"action":"subscribe","quotes":["AAPL"]}
{"action":"subscribe","quotes":["AAPL","MSFT"]}
Success response:
< [{"T":"subscription","trades":[],"quotes":["AAPL"],"bars":[],"updatedBars":[],"dailyBars":[],"statuses":[],"lulds":[],"corrections":[],"cancelErrors":[]}]
< [{"T":"q","S":"AAPL","bx":"V","bp":141.4,"bs":4,"ax":"V","ap":142,"as":1,"c":["R"],"z":"C","t":"2022-05-26T14:42:29.409855829Z"}]
Beautified version of the response:
After subscribing, you will receive a message that describes your current list of subscriptions.
{
"T": "subscription",
"trades": [],
"quotes": [
"AAPL"
],
"bars": [],
"updatedBars": [],
"dailyBars": [],
"statuses": [],
"lulds": [],
"corrections": [],
"cancelErrors": []
}
Refer to Quote Schema
{
"T": "q",
"S": "AAPL",
"bx": "V",
"bp": 141.4,
"bs": 4,
"ax": "V",
"ap": 142,
"as": 1,
"c": [
"R"
],
"z": "C",
"t": "2022-05-26T14:42:29.409855829Z"
}
Quote Schema
Attribute | Type | Notes |
---|---|---|
T | string | message type, always “q” |
S | string | symbol |
ax | string | ask exchange code (Refer to Exchange Codes section) |
ap | number | ask price |
as | int | ask size |
bx | string | bid exchange code |
bp | number | bid price |
bs | int | bid size |
s | int | trade size |
t | string | RFC-3339 formatted timestamp with nanosecond precision (Displayed in UTC timezone) |
c | array | quote condition |
z | string | tape (Refer to Exchange Codes section) |
Streaming Real-Time 1-Minute Bars
If you want to stream 1-minute bars data for a particular stock or stocks, enter below command from wscat command line:
{"action":"subscribe","bars":[<STOCK_SYMBOL>]}
Where <STOCK_SYMBOL> is a list of one or more stock ticker symbols.
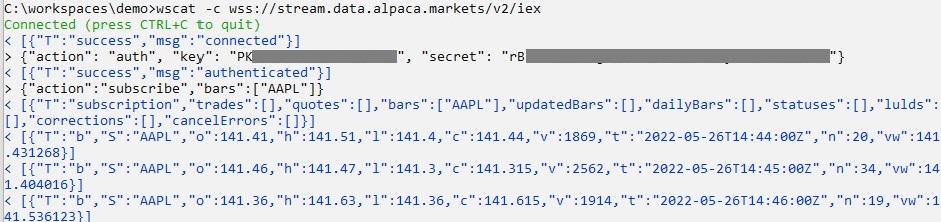
Example:
{"action":"subscribe","bars":["AAPL"]}
{"action":"subscribe","bars":["AAPL","MSFT"]}
Success response:
< < [{"T":"subscription","trades":[],"quotes":[],"bars":["AAPL"],"updatedBars":[],"dailyBars":[],"statuses":[],"lulds":[],"corrections":[],"cancelErrors":[]}]
< [{"T":"b","S":"AAPL","o":141.41,"h":141.51,"l":141.4,"c":141.44,"v":1869,"t":"2022-05-26T14:44:00Z","n":20,"vw":141.431268}]
Beautified version of the response:
After subscribing, you will receive a message that describes your current list of subscriptions.
{
"T": "subscription",
"trades": [],
"quotes": [],
"bars": [
"AAPL"
],
"updatedBars": [],
"dailyBars": [],
"statuses": [],
"lulds": [],
"corrections": [],
"cancelErrors": []
}
Refer to Minute Bar Schema
{
"T": "b",
"S": "AAPL",
"o": 141.41,
"h": 141.51,
"l": 141.4,
"c": 141.44,
"v": 1869,
"t": "2022-05-26T14:44:00Z",
"n": 20,
"vw": 141.431268
}
Minute Bar Schema
Attribute | Type | Notes |
---|---|---|
T | string | message type, always “b” |
S | string | symbol |
o | number | open price |
h | number | high price |
l | number | low price |
c | number | close price |
v | int | volume |
t | string | RFC-3339 formatted timestamp (Displayed in UTC timezone) |
Exchange Codes
List of stock exchanges which are supported by Alpaca.
The tape id of each exchange is returned in all market data requests. You can use this table to map the code to an exchange.
Exchange Code | Name of Exchange |
---|---|
A | NYSE American (AMEX) |
B | NASDAQ OMX BX |
C | National Stock Exchange |
D | FINRA ADF |
E | Market Independent |
H | MIAX |
I | International Securities Exchange |
J | Cboe EDGA |
K | Cboe EDGX |
L | Long Term Stock Exchange |
M | Chicago Stock Exchange |
N | New York Stock Exchange |
P | NYSE Arca |
Q | NASDAQ OMX |
S | NASDAQ Small Cap |
T | NASDAQ Int |
U | Members Exchange |
V | IEX |
W | CBOE |
X | NASDAQ OMX PSX |
Y | Cboe BYX |
Z | Cboe BZX |
References:
The Official documentation of WSCat is here https://www.npmjs.com/package/wscat
From Alpaca Streams API https://alpaca.markets/learn/streaming-market-data/
Subscription Plans https://alpaca.markets/docs/market-data/#subscription-plans
Market Data API Reference https://alpaca.markets/docs/api-references/market-data-api/